MAP DISPLAY WITH GCANVAS
XBit provides an enhanced canvas widget, gcanvas, to display
and edit map objects. At the level of Tcl API, a gcanvas can be considered
an extenstion of the original Tk canvas widget. It supports all the
generic item types and canvas commands that are implemented by the original
Tk canvas. In addition, a gcanvas has its unique features, including
scalable viewport, polygon with holes, text rotation and rescale, scalable
image item, graphic symbol, group item and map object. To support
antomatically label display, gcanvas provides commands for polygon centroid
and line orientation calculations. A gcanvas greatly optimizes its
performance in displaying large maps by using specialized map objects and
floating items.
The following scripts show how to create a polygon with holes and how
to automatically draw line-orientated and polygon-centroided text strings.
set canv .canv
gcanvas $canv -bg #DBDBAB
pack $canv -expand 1 -fill both
font create f0 -family Times -slant roman
# draw a line
set id [$canv create line 10 10 40 40 80 60 120 80 160
90 180 100 240 140 240 220 -width 3]
# draw text strings along the line
foreach pindex {0.25 .45 0.68 0.9} {
foreach {angle x y} [$canv langle
$id $pindex] break
$canv create gtext $x $y -text Text
-font f0 -anchor s -escapement $angle
$canv create gtext $x $y -text Text
-font f0 -anchor n -escapement $angle
}
# create a polygon with two holes
set id [$canv create polygon 20 20 40 10 80 15 100 20
120 25 160 30 \
190 40 210 80 160 200 110 220 60 200 30 180 15 150 \
40 80 80 100 100 90 110 50 80 30 40 35 \
110 110 150 115 170 100 180 65 140 40 120 50 \
{13 6 6} -fill green -outline red]
# draw a text string at the polygon centroid
set centroid [$canv centroid $id]
set x [lindex $centroid 0]
set y [lindex $centroid 1]
$canv create text $x $y -text "Polygon With Holes" -anchor
n
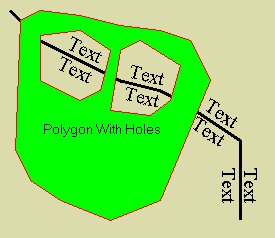
The following scripts show how to create and display a map with a gcanvas
widget, image and map (shape) objects.
# Creating one image object, globe and
two map objects, contries and us (United States).
# All are in longitude and latitude coordinate system
{180W, 90N, 180E, 90S}
image create gimg globe -file globe.jpg -comb
1/2/3 -pal 8/8/4 -x0 -180 -y0 90 -dx 0.5625 -dy 0.5625
shape.create contries -file cntry94.shp
shape.create us -file states.shp
# Creating a gcanvas widget, defining the positive y pointing
toward North and
# setting proper zoom factors and viewport coordinate
origin.
gcanvas .map -mx 1.5 -my -1.5 -viewx -180 -viewy
90 -highlightthickness 0
pack .map -expand 1 -fill both
# Creating one scalable image item and two object items
and display them in the canvas.
.map create image 0 0 -image globe -scalable
1
.map create object 0 0 -object contries
-outline red
.map create object 0 0 -object
us -outline
green

# Choosing a viewport for a region of interest.
.map config -mx 8 -my -8 -viewxorigin -128 -viewyorigin
53

Scalable
Viewport. A gcanvas has a scalable viewport that
pans, flips and magnifies its graphic display without changing the coordinates
of the contained items. Following is a typical viewport specification
of a gcanvas:
gcanvas create gcanvPath
-mx mx -my my -xvieworigin xo
-yvieworigin yo
Options -mx and -my specify maganification factors in x axis
and y axis respectively. A negative magnification factor reverses
the direction of the correspondent coordinate axis. This feature
is very useful since in many cases, a map's y axis points to north, which
is opposite to the direction of the default canvas' y axis. In addition,
a gcanvas item may choose a proper value for an option of -scalable
to decide if it should rescale its size when the viewport's magnification
factors are changed. Options -xvieworigin and -yvieworigin
specify the origins used to convert world coordinates to viewport coordinates.
The translation equations are
xViewport = (xWorld - xo) * mx - xOrigin
yViewport = (yWorld - yo) * my - yOrigin
where xOrigin and yOrigin are controled by other gcanvas commands such
as xview, yview and scan for scrolling canvas window.
Polygon
with Holes. A polygon item of a gcanvas supports
multi-polygon specification. This is done by extending the coordinate
list to include both even and odd number of items. Following is a
typical multi-polygon item specification:
gcanvasPath create polgyon x0
y0 x1 y0... xn yn [{n0 n1... nk}]
If a coordinate list consists of an even number of values, it is considered
a single polygon coordinate specification, which is compatible to the polygon
type of the original Tk canvas. If a coordinate list has an odd number
of items, the last item is considered a polygon vertex definition
list consisting of one or more integers. Each integer defines the
number of vertices of a polygon sequencially specified in the previous
coordinate list. In addition, if a polygon's vertices are specified
in a clock-wise order, it is considered as a normal polygon. Otherwise
it is considered as a hole within another polygon.
Text
Rescale and Rotation. A gcanvas supports a scalable
text
item, which will change its size when the viewport's magnification factors
are changed. To prevent a text string from changing its size, the
value of the option -scalable should be set to 0. In
addition to a text item type, a gcanvas provides a gtext
item type with three more options, -escapment, -orientation
and -aspectratio. Following is a typical gtext
item specification with a 30 degree rotation angle and 0.6 aspect ratio:
gcanvasPath create gtext x y
-texttext -escapement 30.0 -aspectratio
0.6 -orientation 0.0
Option -escapement specifies the angle between the text string's
escapement vector and x axis. Option -orientation speicfies
the angle between each character's base line and x axis. Option -aspectratio
specifies the ratio between a character's width and height. In Windows
NT/2000/XP, when a gcanvas option -graphicsmode is set to 1 (i.e.,
Windows advanced graphics mode), option -escapement can be specified
independently of options -orientation. When a gcanvas option -graphicsmode
is set to 0 (i.e., Windows compatible graphics mode), option -escapement
specifies both the escapement and orientation. In Windows 95/98/Me
the only available Windows graphics mode is compatible mode. A gcanvas
option -graphicsmode does not have any effect and nor does a gtext
item option -orientation. Option -escapement specifies
both the escapement and orientation.
Scalable
Image Item. A gcanvas supports image items as a
Tk canvas does with additional options -scalable and -magnifycommand.
These two options make an image item become automatically rescaled with
gcanvas options -mx and -my. To make a scalable image
item, option -scalable should be configured as "1". Option
-magnifycommand specifies a call back Tcl command imageMagnifyCommand
to magnify the image according to values of gcanvas options -mx
and -my. The call back command must have the following prototype:
imageMagnifyCommand mx my
where mx and my will be appended to imageMagnifyCommand
when it is invoked. A default call back command is provided for an
image item of gimg type, which is able to translate and zoom the
image consistently to its map coordinates and scale.
Symbol
Item. A symoble item is specified with an anchor
point (x, y) and can be used to draw point related symbolic graphics.
A symbol item includes three different components, text, symbol and marker.
While the text component can be placed around the anchor point, the symbol
and marker are centered at the anchor point. Following is a typical
symbol
item specification:
gcanvasPath create symbol x
y -text symbolText -symboltypesymbolType
-markertypemarkerType
Option -text specifies a text string to be placed around the anchor
point (x,y). Option -symboltype specifies a type of
symbol. The currently supported symbol types are oval, rectangle,
diamond
and crosshair. Option -markertype specifies a type
of symoble marker. The currently supported marker types are
point
and cross.
Group
Item. A group item is a container to combine other
gcanvas items. It can be used to draw customized map legends or decorations
for map annotation. Following script creates a group item at an anchor
point (x, y) and adds an oval and a rectangle into it:
set id [gcanvasPath create
group x y]
set gid0 [gcanvasPath group
$id
oval
20
20]
set gid1 [gcanvasPath group
$id
rectangle
-20
-10 -40 10]
When adding an item into a group item, the coordinates of the added item
are relative to the anchor point of the group item and the returned value
is a local item id. Following script deletes the added rectangle
and reconfigurates the added oval:
gcanvasPath group $id delete
$gid1
gcanvasPath group $id itemconfig
$gid0
-fill
red
Other commands of a group item are list, delete and coord
for respectively listing child items, deleting a child item and returning/changing
coordinates of a child item.
Object
Item. An object item is used for a gcanvas to access
to XBit map objects that encapsulate geographic map data sets such as shape
(.shp) files. Following script creates an object item:
gcanvasPath create object x
y -object xbitMapObject
XBit map objects have three types: POINT, LINE and POLYGON. Each
map object can contains hundreds and thousands of components packed in
binary data bits. It is very efficient to display a geographic map
encapsulated in XBit map objects. A gcanvas is not only a tool for
map display but also a tool for map edit. A gcanvas can disassemble
a map object into primitve canvas items such as symbols, lines and polygons
so that they can be edited and then reassembled back into a new map object.
Following Tcl commands respectively disassemble an object into primitive
items and assemble specified items into an object
gcanvasPath disassemble xbitMapObject
gcanvasPath assemble xbitMapObject type
id...
Optimized
Display. To efficiently display graphics,
a gcanvas uses a cached double-buffer to reuse most of the displayed contents
in a window update. A gcanvas can minimize graphic resource allocation
and setup by using specialized shape objects such as line and polygon layers
in map display. In addition, a gcanvas can configure a dynamic item
(i.e., an item with changing size and coordinates) as floating so that
its update will not damage the underneath contents in the cached double-buffer.
Therefore, displaying dynamic items over sophisticated map presentation
can be greatly optimized.
[HOME][CONTENTS][DOWNLOAD] |
|